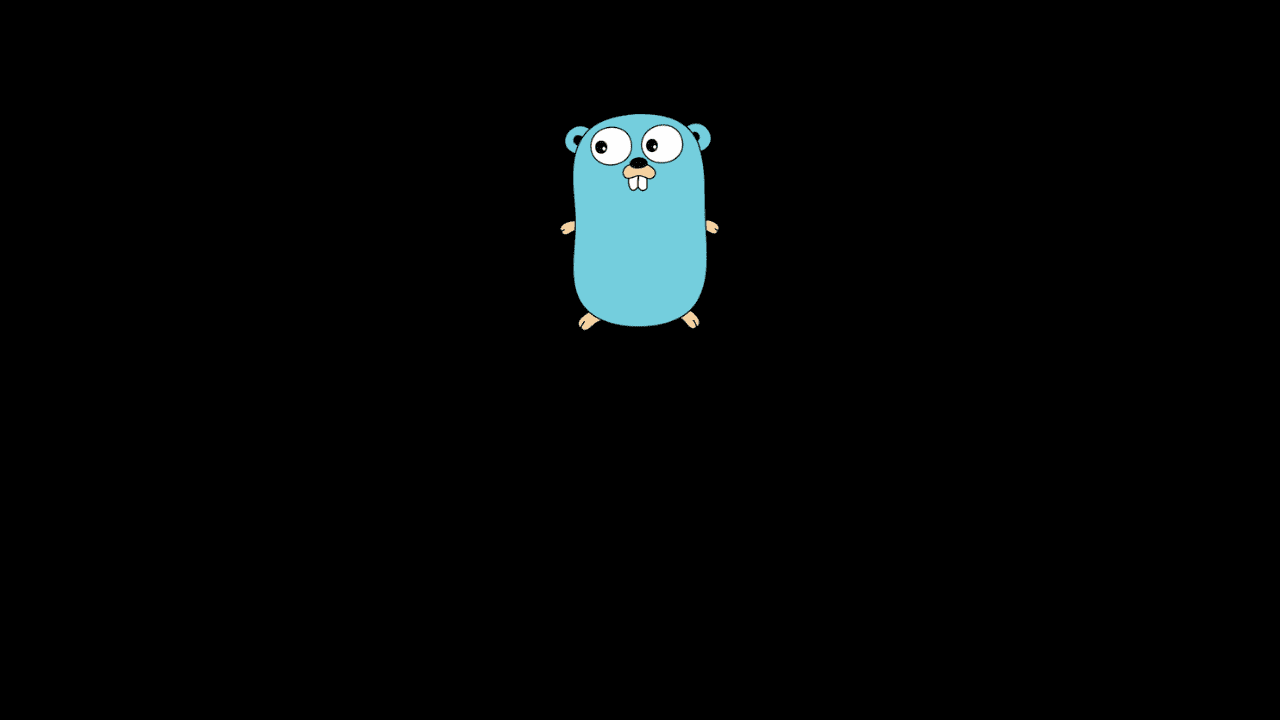
Learn How to use strings in Golang
In this article, you are going to learn about how to use strings in the Go language.
In Go language, strings are immutable which means it is a read-only slice of bytes. Once you have declared a string you will not be able to change it in the Go language. This makes strings different from other programming languages. In Go language, you can use both (“”) double-quotes and (“) backticks as a string literal. Let’s see both of them one by one in the following sections.
When you need to apply escape characters in a string you may use double-quotes. It is widely used in Go language programming languages. See the below code example:
package main
import "fmt"
func main() {
var MyString string
MyString = "Good\\nMorning!"
fmt.Println("My String : ", MyString)
}
/*
Output:
My String : Good
Morning!
*/
Here, you can notice that we have used \n as an escape character into our string and the morning is printed in a new line.
If you need to span multiple lines in the regular expression or Html you may use backticks as a string literal. It is also known as raw literal. It avoids escaping characters. Let’s see the below code example:
package main
import "fmt"
func main() {
var MyString string
MyString = `Good\\nMorning!`
fmt.Println("My String : ", MyString)
}
/*
Output:
My String : Good\\nMorning!
*/
Here, We have used escape characters so it should print in two separate lines as before. But because of using backtick literal it prints in a single line and avoid the escape character.
Sometimes you need to know the length of a string. You can do that easily in the Go language. See the below code example:
package main
import "fmt"
func main() {
var MyString string
MyString = "Good Morning!"
StringLength := len(MyString)
fmt.Println("My String : ", MyString)
fmt.Println("My String length is : ", StringLength)
}
/*
Output:
My String : Good Morning!
My String length is : 13
*/
Here, we have used len() function to get the length of a string.
In the Go programming language, Strings are nothing but a slice of bytes. You can access each byte of a string in the Go language. See the below code example to get things more clear:
package main
import "fmt"
func main() {
str := "Good Morning!"
fmt.Println([]byte(str))
}
// Output: [71 111 111 100 32 77 111 114 110 105 110 103 33]
This is all about strings in the Go language and different ways of printing them.