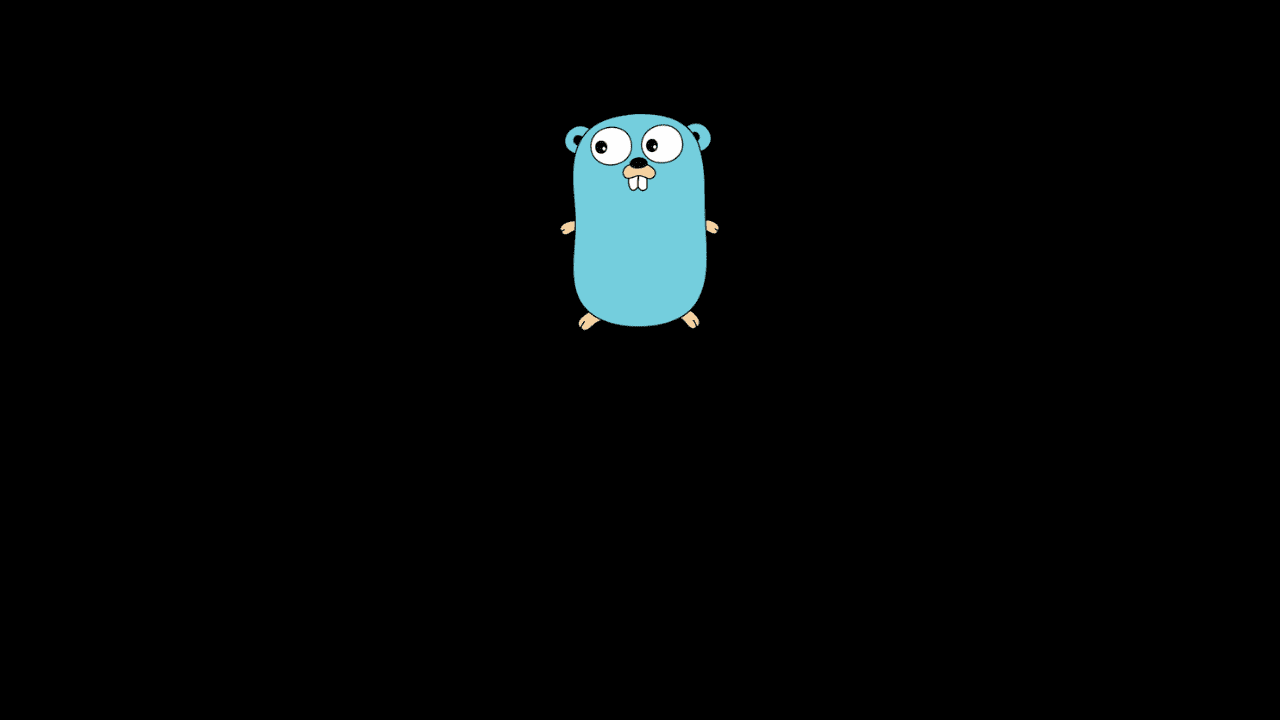
Learn How to read input from STDIN in Golang
In this article, you are going to learn about how to read input from STDIN in the Go language.
In many programming languages, you can read data from a console and see the output. The Go language is not out of that. You can also read input from STDIN. To do so, you need to import few essential packages – “bufio”, “fmt”, “os”. The “bufio” package helps you to read a bunch of characters at a single time and the “fmt” package provides you functions that can read and write from I/O. Finally the “os” package lets you perform operating system functionality.
In the Go language, you may read input from STDIN two ways. Like read a single character or read multiple lines. Let’s see how you can read a single character from STDIN in the below code example:
package main
import (
"bufio"
"fmt"
"os"
)
func main() {
fmt.Printf("Enter a single Character: ")
reader := bufio.NewReader(os.Stdin)
char, _, err := reader.ReadRune()
if err != nil {
fmt.Println(err)
}
fmt.Println(char)
}
Output:

You can see that when we input a single character, it gives us the ASCII value of that particular character. In our case, we have given “D” as input and the ASCII value for D is “68” has been shown.
If you want to read multiple characters from STDIN in the Go language, it is also possible. Let’s see the below code example, where we have written a program. The program asks for your name and you may write your name in the console.
package main
import (
"bufio"
"fmt"
"os"
)
func main() {
fmt.Printf("Enter Your Name: ")
var reader = bufio.NewReader(os.Stdin)
name, _ := reader.ReadString('\n')
fmt.Println("You
Output:
Here, we can see that our program has been completed successfully and this is how you can take input from STDIN in the Go language.