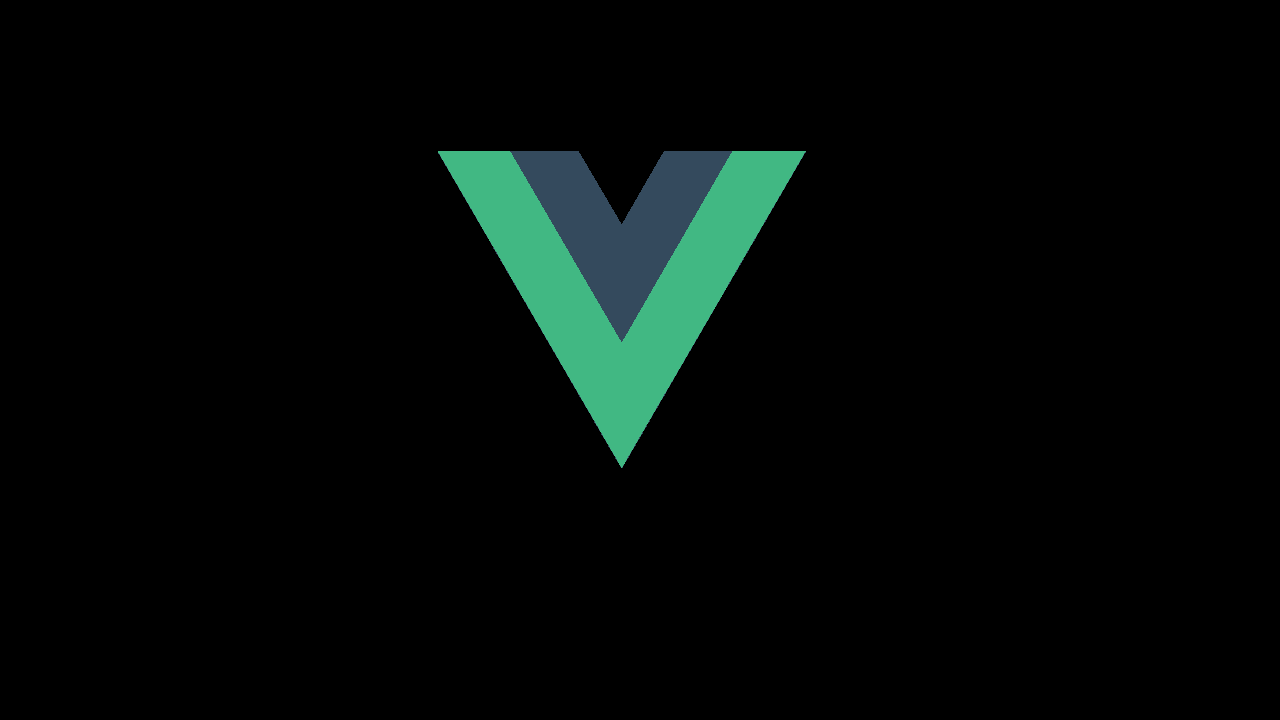
How to use img src in VueJs
In this article, you are going to learn about how to use img src in VueJs.
Vue is a modern JavaScript framework that is widely used in building user interfaces and progressive web applications. With Vue, you can do lots of eye-catching things but here I will show you how you can work with img src. The best way to understand this things is work with an example.
Let’s imagine we want to see students’ names along with their pictures. Here, in the below example we will demonstrate our imagination and will show students pictures by using imr src. See the below code example:
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="<https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/css/bootstrap.min.css>" />
<link rel="stylesheet" href="style.css">
<title>Use Img Src</title>
</head>
<body>
<form id="studentForm">
<div>
<div id="student">
<table class="table table-bordered text-center">
<tr>
<td> Student Name </td>
<td>Student Picture </td>
</tr>
<tr v-for="students in StudentInfo">
<td>{{students.Name}}</td>
<td>
<img v-bind:src="students.Url" width="100px" height="120px" alt="">
</td>
</tr>
</table>
</div>
</div>
</form>
<script src="<https://cdn.jsdelivr.net/npm/vue@2.6.14/dist/vue.js>"></script>
</body>
</html>
Here, we add the bootstrap file to make things simple and also link vue CDN file. We took help of v-bind:src to get the URL from Vue file. Let’s implement out VueJs code to make things work:
VueJs:
var app = new Vue({
el: '#student',
data: {
StudentInfo: [
{
"id": "1",
"Name": "Alina",
"Url": "img/alina.jpg"
},
{
"id": "2",
"Name": "Deven",
"Url": "img/deven.jpg"
},
{
"id": "3",
"Name": "Alex",
"Url": "img/alex.jpg"
}
]
}
})
Here, we have linked our image URL . In our case we are using our locally saved images. You may you online sources or your own downloaded images. All you need to do just linked that name of the images. Let’s see our final outcome in the below:
Output:

You can see that we have successfully implemented our functionality. The student’s names along with their picture is shown in the browser. This is how you can use img src and work with it in VuejS.
Important: If you are using vue-cli you need to be careful because here everything is processed as a module including images. In such cases, you need to use require for the relative path