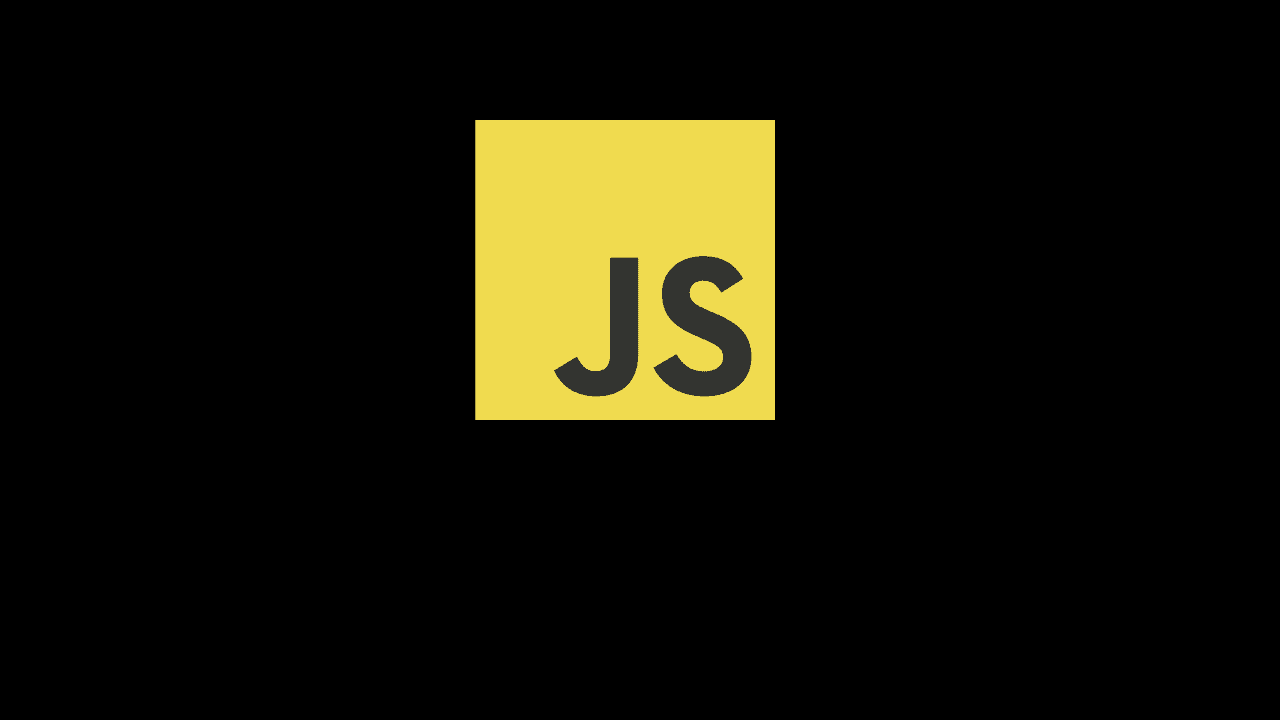
How to Validate Email in Javascript
Posted on: March 06, 2021 by Ariessa Norramli
In this article, you will learn how to validate an email in Javascript.
Validate Email
In order to validate email, you can use the regular expression
and test()
method.
var a = "test@gmail.com";
var b = "test.example.com";
var c = "A@b@c@example.com";
function validateEmail(email) {
// Regular expression for email search pattern
var re = /^[^\s@]+@[^\s@]+$/;
// If email's pattern is found in variable re
if (re.test(email)) {
// Display valid email message
console.log(email + " is a valid email address");
}
// Else if email's pattern cannot be found in variable re
else {
// Display invalid email message
console.log(email + " is an invalid email address");
}
}
validateEmail(a);
// => "test@gmail.com is a valid email address"
validateEmail(b);
// => "test.example.com is an invalid email address"
validateEmail(c);
// => "A@b@c@example.com is an invalid email address"
Note: The regular expression
is a sequence of characters used as a search pattern. The test()
method functions by testing for a match in the supplied string.
Share on social media
//