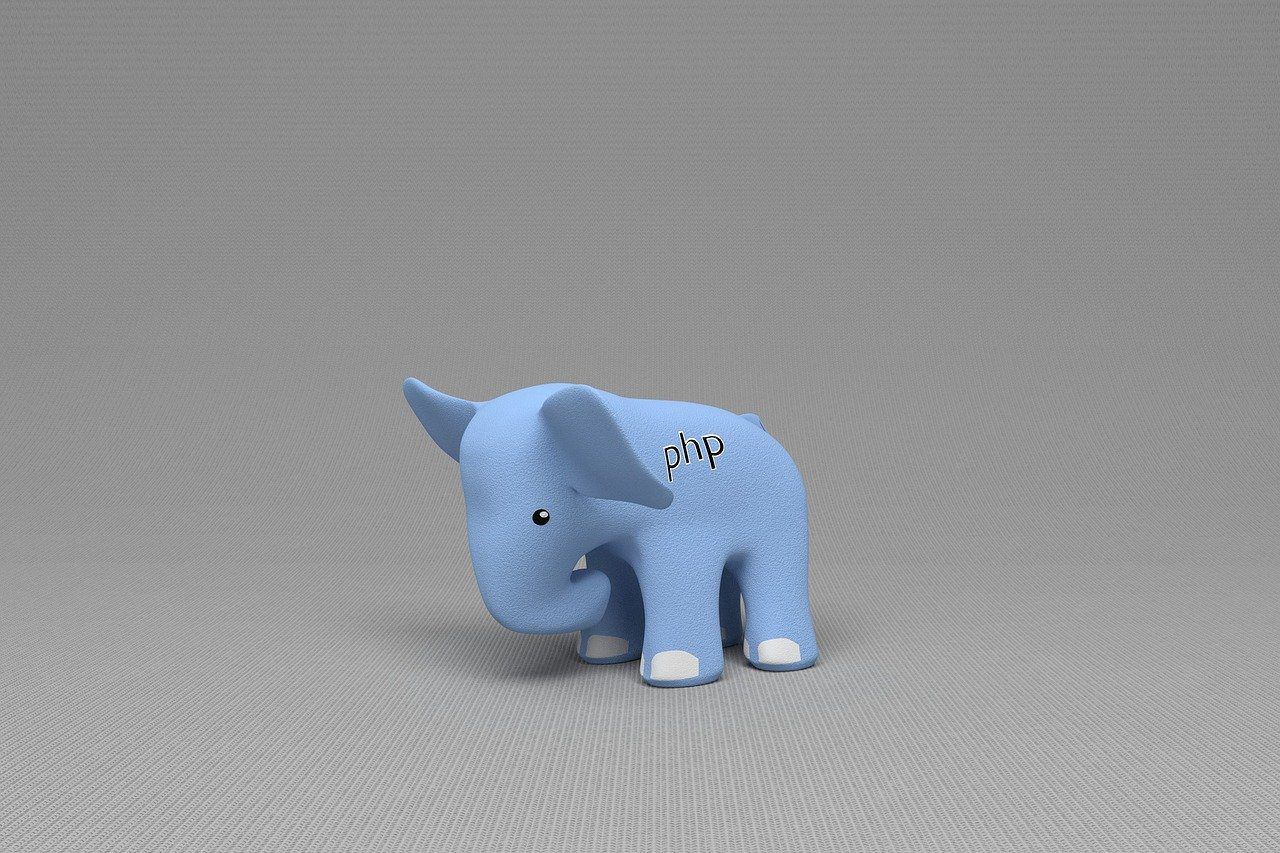
How to Upload Multiple Files in PHP
Posted on: March 06, 2021 by Ariessa Norramli
In this article, you will learn how to upload multiple files in PHP.
Upload Multiple Files
In order to upload multiple files, you can use the move_uploaded_file()
method.
<html>
<body>
<form method='post' action='' enctype='multipart/form-data'>
<!-- Input of type multiple files -->
<input type="file" name="file[]" id="file" multiple>
<!-- Upload button -->
<input type='submit' name='submit' value='Upload'>
</form>
<?php
// Path to store uploaded file
$path = "C:/xampp/htdocs/";
// If the submit button is pressed
if(isset($_POST['submit'])){
// Count total files
$fileCount = count($_FILES['file']['name']);
// Iterate through the files
for($i = 0; $i < $fileCount; $i++){
$file = $_FILES['file']['name'][$i];
// Upload file to $path
move_uploaded_file($_FILES['file']['tmp_name'][$i], $file);
}
}
?>
</body>
</html>
Note: The move_uploaded_file()
method functions by moving an uploaded file into a new location.
Share on social media
//