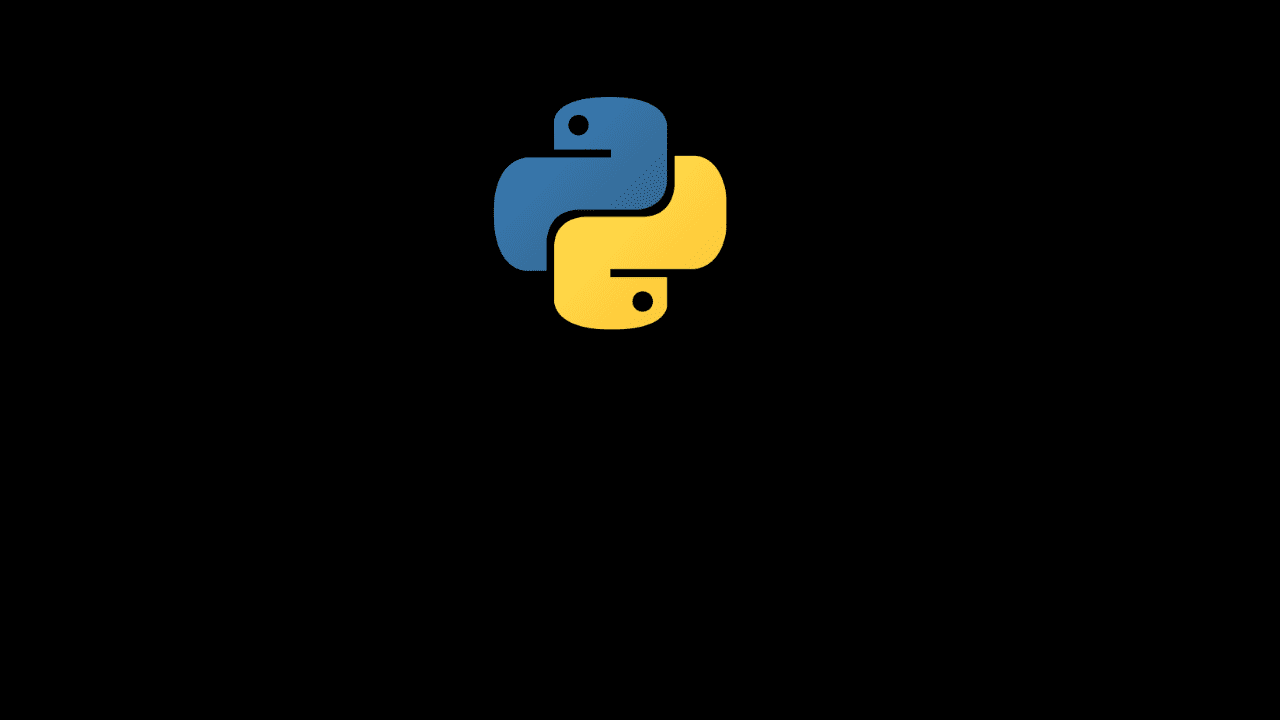
How to Set Column As Index in Python
Posted on: February 25, 2021 by Ariessa Norramli
In this article, you will learn how to set a column as an index in Python.
Let’s say you have a DataFrame of food.
# Import pandas module
import pandas as pd
# Create DataFrame
df = pd.DataFrame({"Name": ['Pizza', 'Burger', 'Waffle'],
"Price ($)": [10, 5, 6],
"Availability": [True, False, True],
"Delivery": [True, True, True],
"Pickup": [True, True, False]})
Set Column As Index in Python
In order to set a column as an index, you can use the DataFrame.set_index()
method.
# Import pandas module
import pandas as pd
# Create DataFrame
df = pd.DataFrame({"Name": ['Pizza', 'Burger', 'Waffle'],
"Price ($)": [10, 5, 6],
"Availability": [True, False, True],
"Delivery": [True, True, True],
"Pickup": [True, True, False]})
# Set column "Name" as index column
df.set_index("Name", inplace = True)
# Display DataFrame
print(df)
Note: The DataFrame.set_index()
method functions by setting the supplied column name as index column. The inplace
parameter functions by making the changes if the supplied column name exists in DataFrame.
Share on social media
//