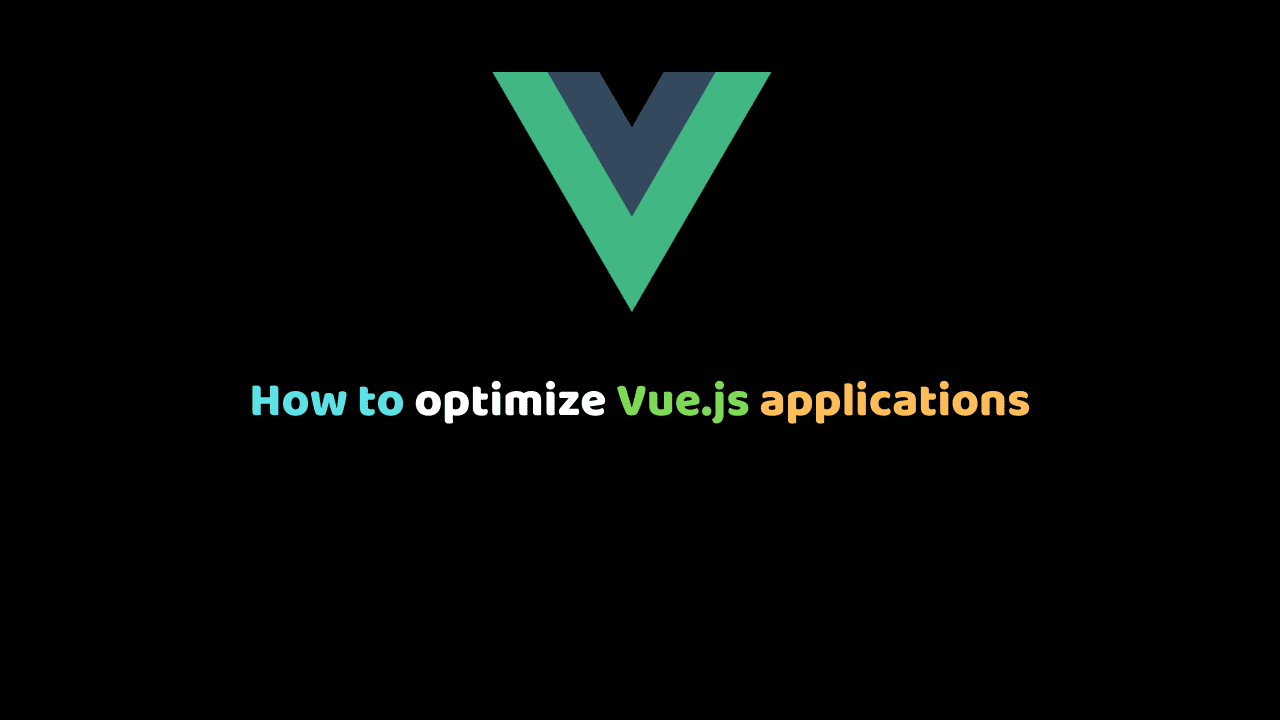
How to optimize Vue.js applications
Just like every other application out there that grows in size, Vue.js applications also deteriorate in performance as the application grows. In this article, we’ll briefly discuss some of the techniques in optimizing Vue.js applications.
Let’s get cracking.
The ways to optimize a Vue.js application
Here are a few tips to make your Vue applications faster and lighter:
Lazy load Images
If you happen to be working on a project that deals with a lot of images, say an e-commerce store for example. A general rule of thumb is that you lazy load the images. There is an NPM module for Vue that makes lazy loading images easy: vue-lazyload.
Just by installation and setting up some of the configurations (or you could use the default), you’re good to go.
npm i vue-lazyload -S
And in main.js
file:
// in main.js
import Vue from 'vue'
import App from './App.vue'
import VueLazyload from 'vue-lazyload'
Vue.use(VueLazyload)
new Vue({
el: '#app',
components: {
App
}
})
We then utilize the module as a directive in our templates:
<ul>
<li v-for="img in list">
<img v-lazy="img.src">
</li>
</ul>
Only use the packages you need
This is another area where bottlenecks begin to surface in our application because of some third-party package. Let’s take Bootstrap as an example, If you only need the responsivity Bootstrap provides you, then there’s no need to even use it at all, yes it might be a drag to write your own CSS but it’s going to be worth it. Bootstrap comes bundled with more features than the responsivity and adding all that redundant code to your application will increase its bundle size (not good).
Lazy load routes
Lazy loading routes is a great way to increase page load speed, it makes code for a specific page load only when the user navigates to that page, this way, the pages are split into separate chunks which makes the initial page load much faster.
// in router.js
import Home from '@/views/Home.vue'; // traditonal imports
const About = () => import('@/views/About.vue'); // dynamic imports
const router = new VueRouter({
routes: [
{ path: '/', component: Home },
{ path: '/about', component: About }
]
})
It is also possible to group route components into the same chunk, this is a great idea when pages are related by content and the user will most likely navigate to the related pages. These could be components nested under the same route.
// in router.js
import Home from '@/views/Home.vue'; // traditonal imports
import User from '@/views/User.vue';
const About = () => import('@/views/About.vue'); // dynamic import
const router = new VueRouter({
routes: [
{ path: '/', component: Home },
{ path: '/about', component: About },
{ path: '/user/:id', component: User,
children: [
{
path: '/settings',
component: () => import(/* webpackChunkName: "user" */ '@/views/UserSettings')
},
{
path: '/articles',
component: () => import(/* webpackChunkName: "user" */ '@/views/UserArticles')
}
]
}
]
})
Using the comment syntax /* webpackChunkName: "user" */
, webpack will bundle any module with the same chunk name together in the same chunk, in the case the user
chunk.
Do not clutter the store
Yes, do not add data that will be used by less than 3 components to the Vue store. It’s not response from an API that needs to be stored with Vuex. You don’t want to have a large store filled with data that doesn’t really need to be there.
TL;DR
Just in case you don’t stick around for the entire article, here’s a summary for you to optimize Vue.js application
- If your app has a lot of images, lazy load them.
- You should only use the packages you need.
- Lazy load routes that won’t be needed on page load and group components by chunks if possible.
- Do not place unnecessary data in the store.