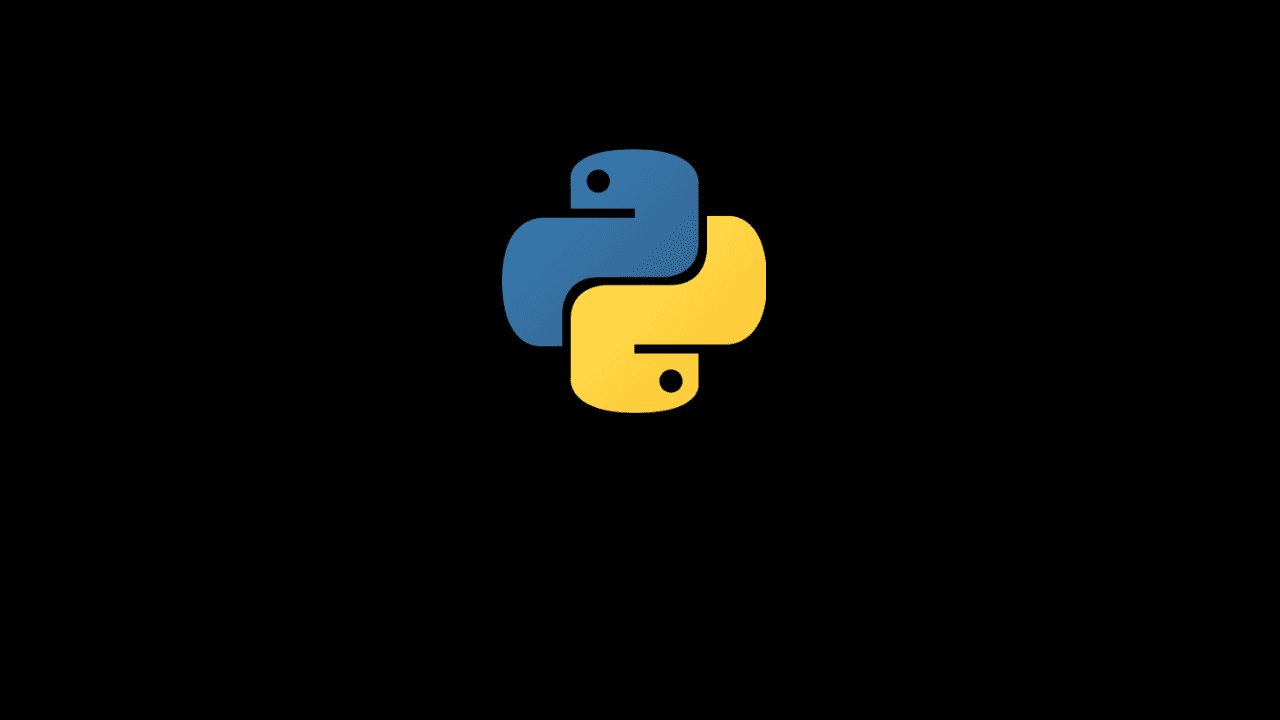
How to Drop Rows With Missing Values in Python
Posted on: February 24, 2021 by Ariessa Norramli
In this article, you will learn how to drop rows with missing values in Python.
Let’s say you have a DataFrame of food with two columns, “Name” and “Price ($)”. One of the data in column “Price ($)” is missing and therefore labelled as pd.NaT. In the pandas
module, NaT is used to represent missing values.
# Import pandas module
import pandas as pd
df = pd.DataFrame({"Name": ['Pizza', 'Burger', 'Waffle'],
"Price ($)": [10, pd.NaT, 6]})
In order to drop rows with missing values, you can use the DataFrame.dropna()
method.
# Import pandas module
import pandas as pd
df = pd.DataFrame({"Name": ['Pizza', 'Burger', 'Waffle'],
"Price ($)": [10, pd.NaT, 6]})
print(df.dropna())
Note: The DataFrame.dropna()
method functions by dropping rows with missing values by default.
Share on social media
//