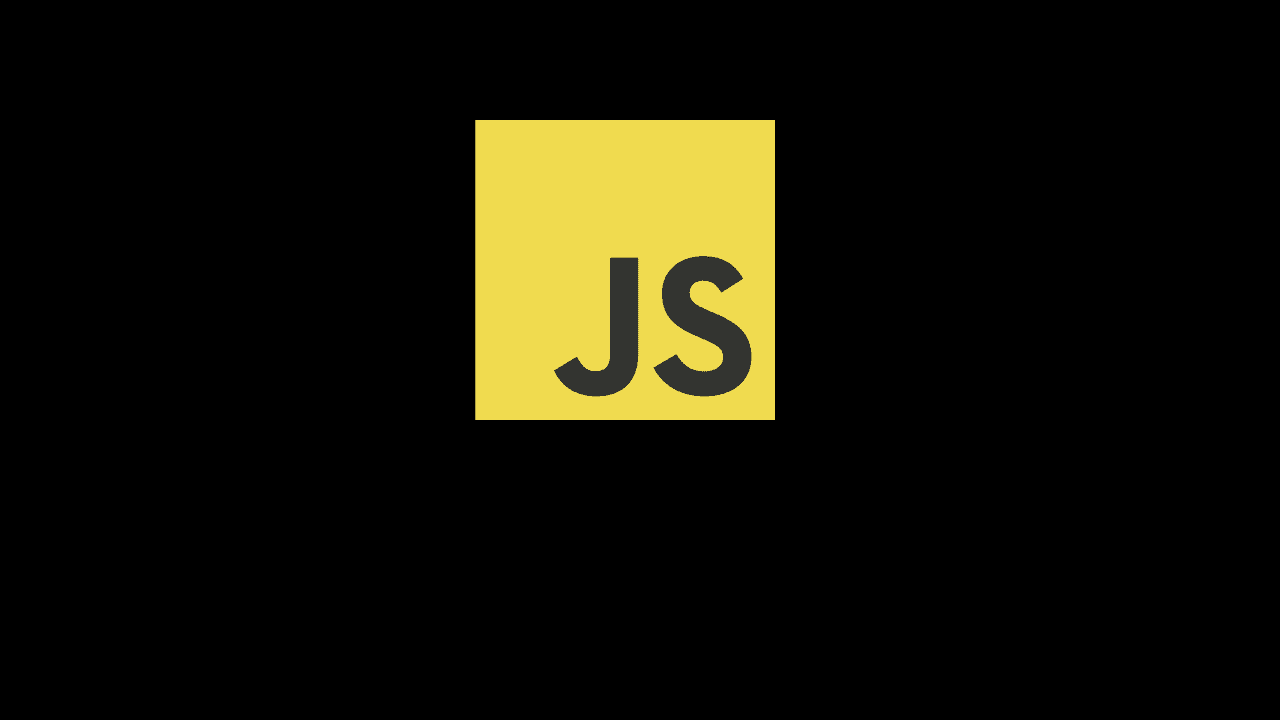
How to Deep Copy Array Using Lodash
Posted on: February 19, 2021 by Ariessa Norramli
In this article, you will learn how to deep copy an array using Lodash.
Let’s say you have an array named ‘a’ with elements ‘1, 2, 3, 4’.
// Import Lodash library
import _ from "lodash";
var a = [1, 2, 3, 4];
In order to deep copy an array using Lodash, you can use the _.cloneDeep()
method.
// Import Lodash library
import _ from "lodash";
var a = [1, 2, 3, 4];
// Create a deep copy of the previous array
var b = _.cloneDeep(a);
// Change the first element of array 'b' to 9
b[0] = 9;
console.log(a);
// => [1, 2, 3, 4]
console.log(b);
// => [9, 2, 3, 4]
Note: The _.cloneDeep()
method functions by creating a fully independent yet exact copy of supplied values.
Share on social media
//