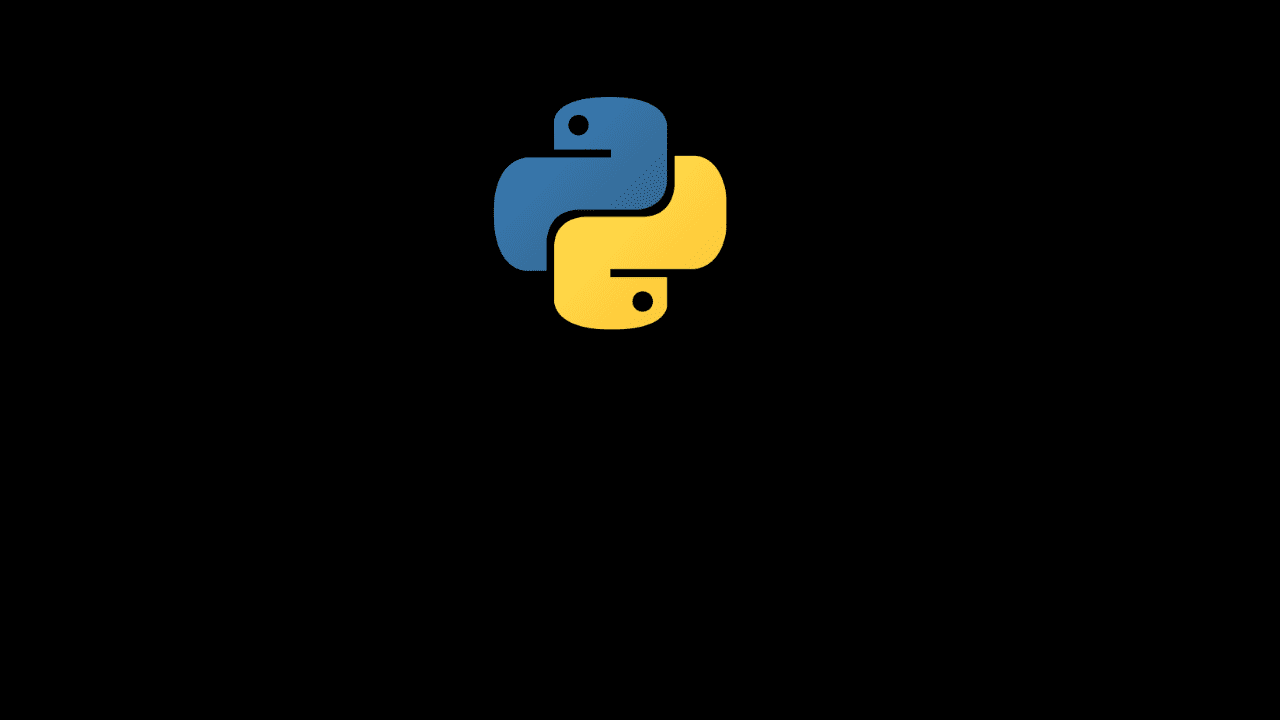
How to Check Data Type of Variables in Python
Posted on: February 22, 2021 by Ariessa Norramli
In this article, you will learn how to check the data type of variables in Python.
Let’s say you have 5 variables.
# A variable named 'a' with value 123
a = 123
# A variable named 'b' with value "codesource"
b = "codesource"
# A variable named 'c' with value False
c = False
# A variable named 'd' with value ["red", "green", "blue"]
d = ["red", "green", "blue"]
# A variable named 'e' with value {"north", "south", "east", "west"}
e = {"north", "south", "east", "west"}
In order to check the data type of variables, you can use the type()
method.
# A variable named 'a' with value 123
a = 123
# A variable named 'b' with value "codesource"
b = "codesource"
# A variable named 'c' with value False
c = False
# A variable named 'd' with value ["red", "green", "blue"]
d = ["red", "green", "blue"]
# A variable named 'e' with value {"north", "south", "east", "west"}
e = {"north", "south", "east", "west"}
print(type(a))
# => <class 'int'>
print(type(b))
# => <class 'str'>
print(type(c))
# => <class 'bool'>
print(type(d))
# => <class 'list'>
print(type(e))
# => <class 'set'>
Note: The type()
method functions by returning the class type of supplied argument.
Share on social media
//